Secure File Transfer with Java SFTP Library | JSCAPE
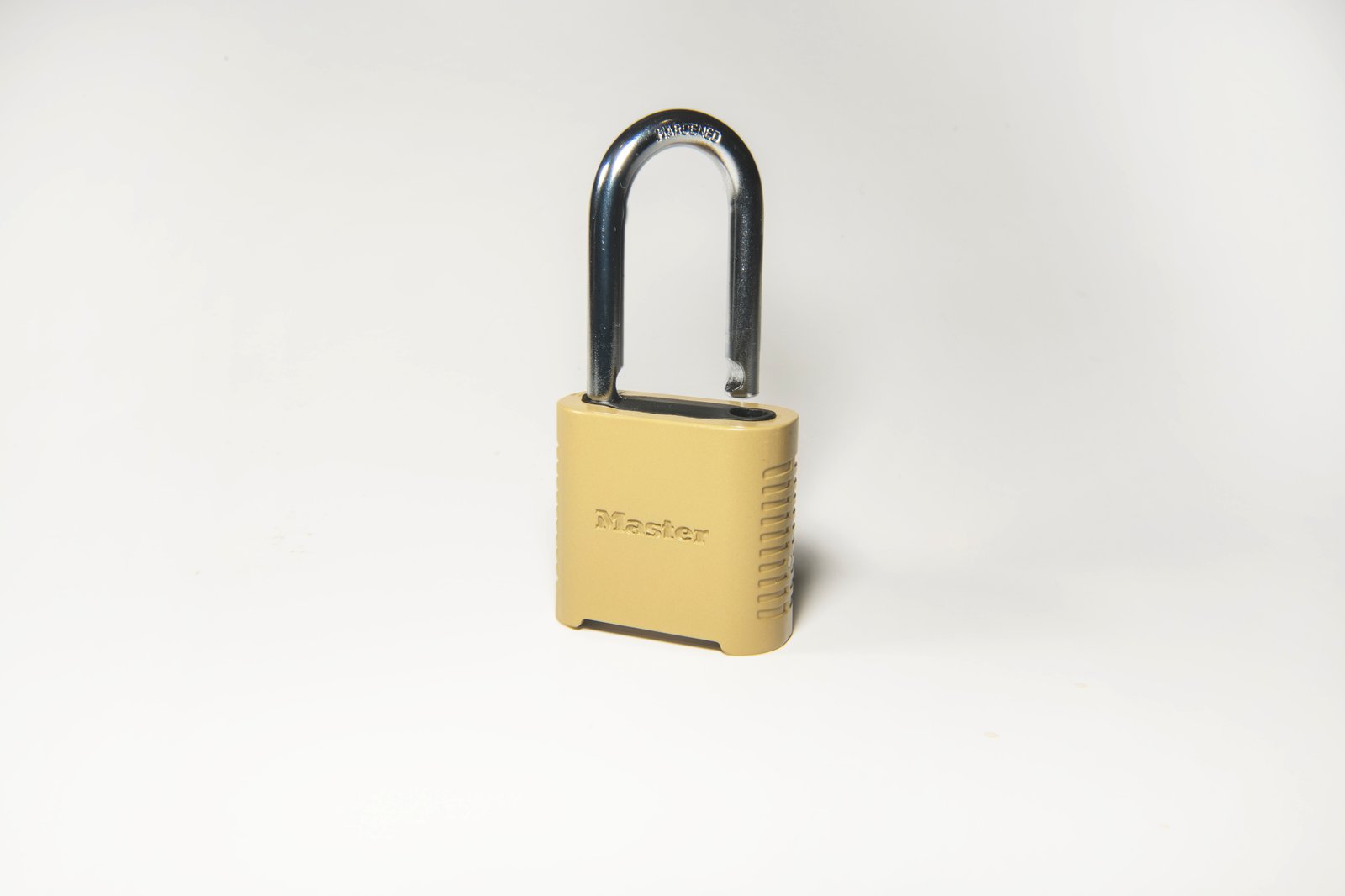
If you're a programmer, you've already learned dozens of TLA's (Three Letter Acronyms). If a phrase has four words, of course, we don't call the acronym an "FLA" because any civilian could understand that. We call it an ETLA (Extended Three Letter Acronym)! In the previous article, you saw how to use JSCAPE's Secure FTP Factory to safely transmit files using the fine ETLA FTPS. This time, we'll show you an even better way to transfer files securely using the even cooler ETLA SFTP. Being able to remember things like this is the mark of a professional programmer.
Actually, SFTP is usually preferred because it uses only one port, typically port 22. FTP and FTPS use port 21 for commands, but then set up a separate data channel (port) each time a directory listing or file transfer is performed. In order to handle several concurrent connections FTP/S may require opening a large port range on the firewall, whereas with SFTP you only have to open a single port.
The methods for creating and using an Sftp object are very similar to the methods you have seen already, which is particularly nice because internally, SFTP doesn't have anything to do with FTPS at all. SFTP was written by an entirely different group, the IETF (Internet Engineering Task Force, another swell ETLA). It makes use of a secure channel set up using the SSH protocol (Secure Shell, a TLA which resolves to only two words. You're feeling more professional already, right?). However, the JSCAPE (not an acronym for anything, thank you) code to upload files is very similar:
File Upload with Java SFTP Library
import com.jscape.inet.sftp.*; import com.jscape.inet.sftp.events.*; import com.jscape.inet.ssh.util.SshParameters; import java.io.*; import java.util.Enumeration; public class SftpUpload extends SftpAdapter { public void doUpload() throws SftpException { String hostname = "ftp.somewebsite.com"; String username = "SmartProgrammer"; String password = "OIMAQT"; // The "Sftp" constructor wants to see an "SshParameters" object, rather than individual Strings. SshParameters params = new SshParameters(hostname,username,password); Sftp ftp = new Sftp(params); // Connect, upload PNG images and release the connection. ftp.connect(); ftp.mupload(".*\\.png"); ftp.disconnect(); } public static void main(String[] args) { try { SftpUpload theUploader = new SftpUpload(); theUploader.doUpload(); } catch(Exception e) { e.printStackTrace(); } }
Naturally, you will need to have a server such as JSCAPE MFT Server that supports this protocol. Also note that the Sftp constructor wants to see an SshParameters object rather than individual strings.
Downloading is almost the same:
File Download with Java SFTP
/* * SftpDownload.java * Simple example of SFTP (SSH-based) file download. */ import com.jscape.inet.sftp.*; import com.jscape.inet.sftp.events.*; import com.jscape.inet.ssh.util.SshParameters; import java.io.*; import java.util.Enumeration; public class SftpDownload extends SftpAdapter { public void doDownload() throws SftpException { // Create an SschParameters object to give to the sftp constructor. // For this example, we also set up th filter as a String. String hostname = "some.website.com"; String username = "SmartProgrammer"; String password = "OIMAQT"; String filter = ".*\\.png"; SshParameters params = new SshParameters(hostname, username, password); // create new Sftp object Sftp ftp = new Sftp(params); // establish connection, download the PNG files and "hang up". ftp.connect(); ftp.mdownload(filter); ftp.disconnect(); } public static void main(String[] args) { try { SftpDownload theDownloader = new SftpDownload(); theDownloader.doDownload(); } catch(Exception e) { e.printStackTrace(); } } }
To sum up, SFTP uploads and downloads are almost the same code as FTP/S, but the SFTP protocol is usually preferred due to it being more firewall friendly.