How To Create Custom Trigger Functions | JSCAPE
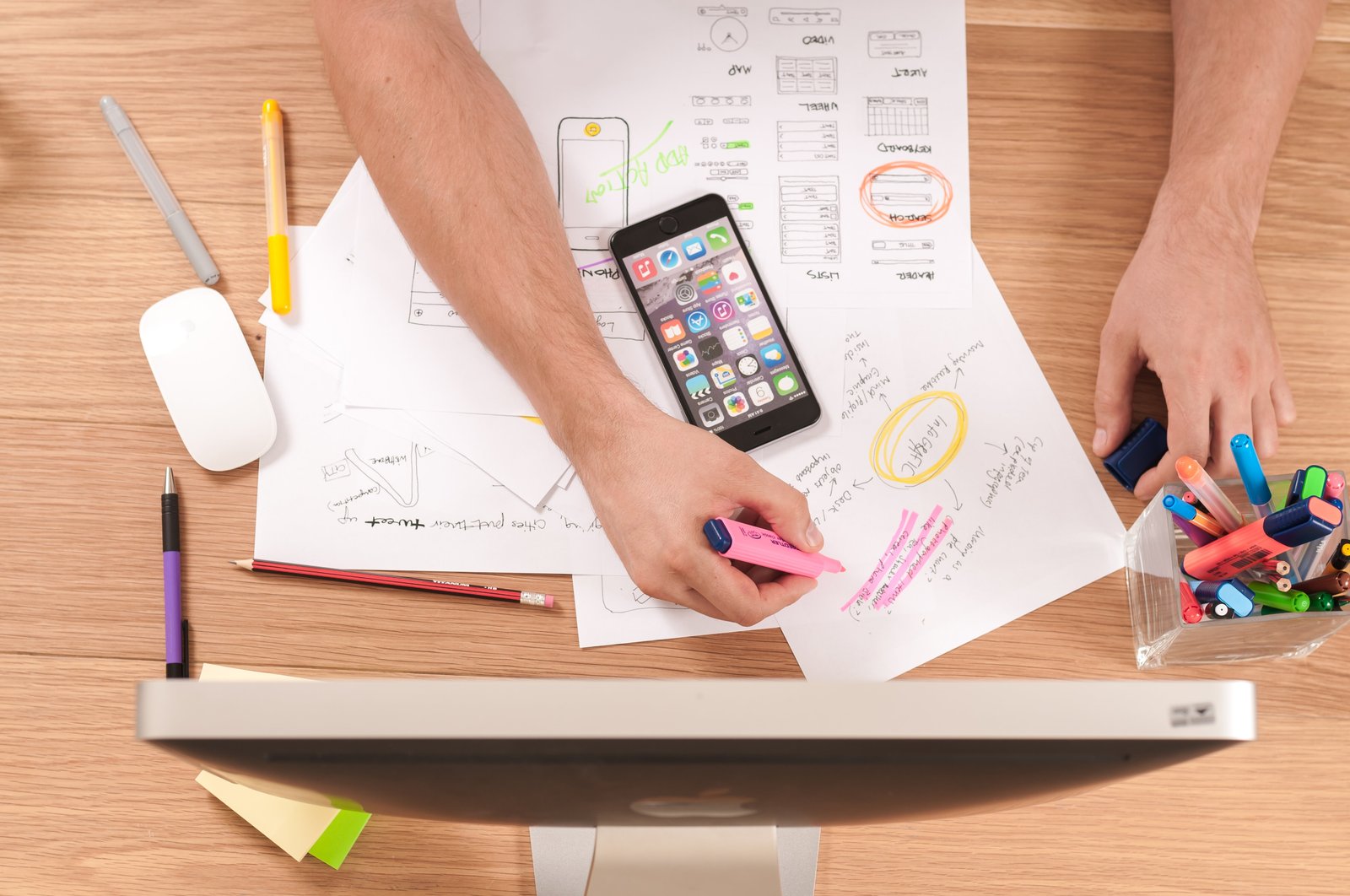
Overview
In the tutorial How To Create Custom Trigger Actions we demonstrated how to create custom trigger actions in MFT Server.
In this tutorial we will discuss Functions, how they are used in Triggers, and how to create your own custom Functions using the extensible API provided in MFT Server.
For most business use-cases you can use the existing functions available in MFT Server. The focus of this tutorial is to address those use-cases where a built-in function is not available to meet your needs.
One of our customers recently asked how to automate a daily scheduled file upload excluding holidays. Scheduling file uploads is pretty straight forward, (See Using time based triggers), but holidays are locale dependent and as of the date of this article there is no module for managing holidays in MFT Server. We realized that this would be a perfect opportunity to create a custom function that does the work for us.
In this tutorial, we will demonstrate how to create and install a simple IsHoliday custom trigger function.
Minimum Requirements
- Java programming experience
- Java IDE
- JDK (Minimum requirements vary based on your version of JSCAPE MFT Server. Consult your documentation for details)
- Experience creating Triggers in JSCAPE MFT Server
Functions vs. Actions
As I started to write this article I asked myself: What is the difference between a Function and an Action? I soon realized that the difference was not immediately apparent. I settled on the definition that in the context of a trigger, Functions are typically used for the transformation and evaluation of event variables, whereas Actions are used to perform a unit of work often using event variables and the results of Functions as inputs.
Still confused? To better illustrate letβs take the simple use-case where anytime a file is uploaded to MFT Server ending with an .ext extension that we want to rename that file using the current timestamp. To begin we will create a trigger that listens for the File Upload event. Next we add a trigger Condition using the EndsWith(LocalPath,".ext") function to check that the event variable containing the filename, LocalPath, ends with the desired extension. Lastly we rename the file using the Rename File action using the CurrentTime function as an input.
Getting Started
The IsHoliday
function we are developing as part of this tutorial will have File
and optional Date
inputs and will return a true
value if the specified Date
is found in the File
,where the File
contains a list of all holidays in the format MM/dd/YYYY
, one per line. Before we fire up our IDE and start looking at source code it may be helpful to understand the basic components of a trigger function.
At it's core a function has zero or more inputs and returns a value upon execution. The inputs to the function are populated at design-time when creating a trigger and may contain static or variable data (i.e event variables) that are executed upon at run-time. The value returned by the function can be any java.lang.Object
instance provided it overrides the toString()
method.
Coding Requirements
- In order to compile your custom function you must include all Java libraries found in the
libs
directory relative to your JSCAPE MFT Server installation in your IDE projectCLASSPATH
. - All custom functions must extend the abstract class
com.jscape.inet.mft.Function
and implement the abstract methods therein, namelygetName()
,getTemplates()
andevaluate(Object[] value)
.
Example Code
Code Analysis
- Lines 1 - 11: Necessary import statements.
- Line 14: Class definition. Must extend abstract class
com.jscape.inet.mft.Function
- Line 16: static variable
pattern
used to define date format used in holiday input file. - Lines 21 - 23: Override
getName()
method. This is the name displayed in Triggers module when using custom functions. - Lines 31 - 36: Override
getTemplates()
method, defining function signatures available for use. These signature definitions, along with a description of the function are included in theHashMap
that is used by MFT Server when populating the list of available functions for use in triggers. In this case there are two functions available, one that accepts an input file operating on the current dateIsHoliday(file)
and a second that accepts both an input file and dateIsHoliday(file, date)
. - Lines 39 - 73: Override
evaluate(Object[] value)
method. This method is responsible for determining whether the input is a holiday and returning eithertrue
orfalse
.
Execution
This is where the magic happens. In the evaluate(Object[] value)
method of your Function
implementation add the code to execute when running this function and the value to return. This is shown in Lines 39 - 73 of the supplied IsHoliday
source code example.
Installation
For custom functions to be made available you must create a JAR archive that contains your custom functions, place it in the libs/ext
directory relative to your MFT Server installation and restart your MFT Server instance. Any third party libraries that your functions depends on should be placed in the libs
directory of your JSCAPE MFT Server installation.
For your function to be recognized you must include a special MANIFEST.MF
file in your JAR archive that identifies those classes that are functions. Below are the contents of the MANIFEST.MF
file used in the IsHoliday
example.
Manifest-Version: 1.0
JSCAPE-Secure-Server-Functions: com.jscape.inet.mft.functions.repo.IsHoliday
If your JAR file has multiple classes that are functions then you may delimit these using commas between class names e.g.
Manifest-Version: 1.0
JSCAPE-Secure-Server-Functions: com.mydomain.Class1,com.mydomain.Class2
To verify that your function has been successfully installed login to the administrative interface and navigate to the Triggers module for your domain. Click on the Functions tab to verify that your newly created function is visible.
Want to try this out? Download the free, fully-functional JSCAPE MFT Server Starter Edition.
Download JSCAPE MFT Server Trial