How to create custom trigger actions
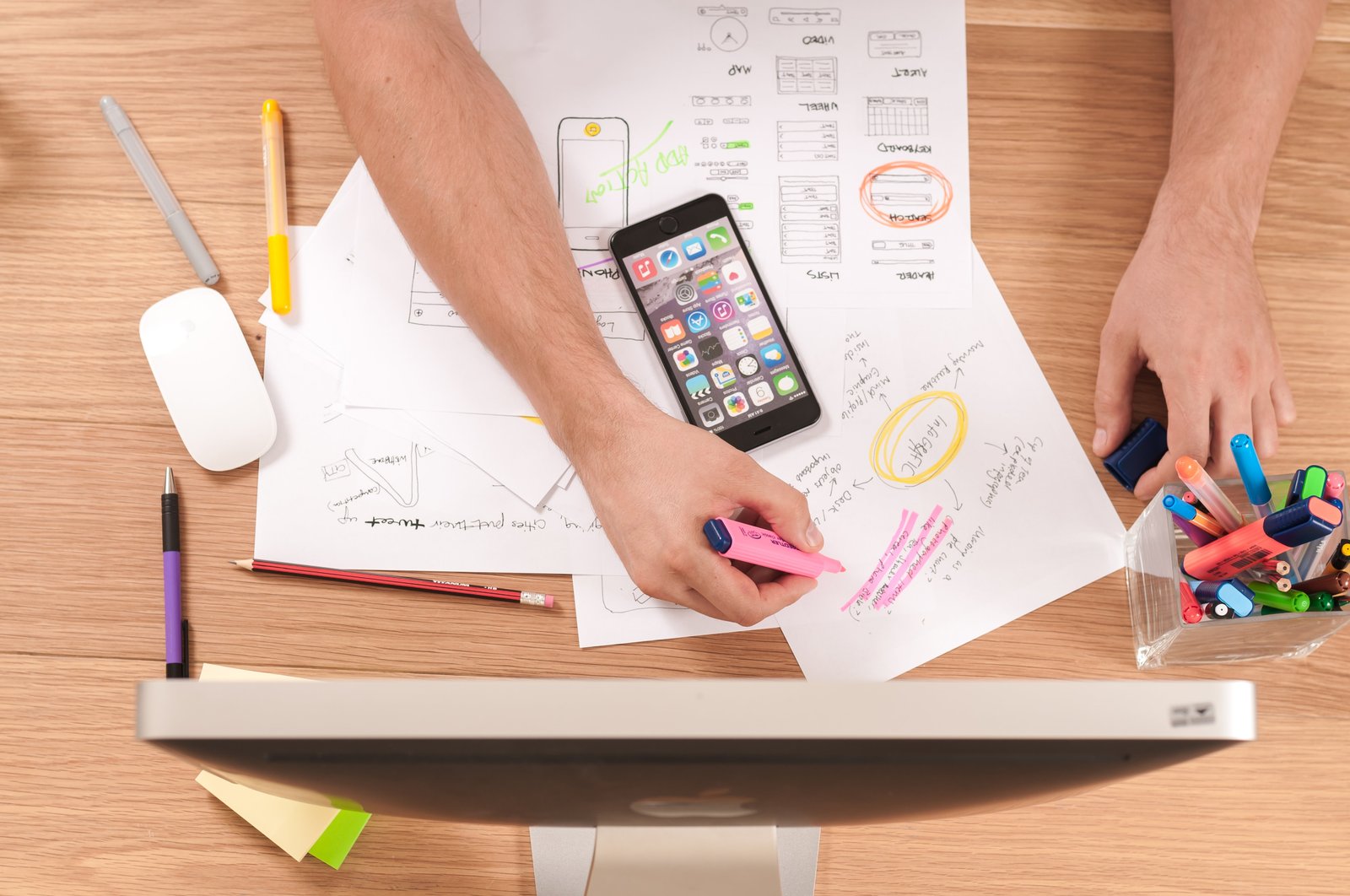
Overview
Triggers are a very powerful feature that allow you to listen for events and respond with actions if conditions are met. For example, whenever a file is uploaded by a certain user you may wish to have this file encrypted and then send an email notification with details of the file transfer.
For most business use-cases you can use the existing actions available in JSCAPE MFT Server. The focus of this tutorial is to address those use-cases where a built-in action is not available to meet your needs.
In this tutorial, we will demonstrate how to create and install a simple HelloWorld custom trigger action for use in JSCAPE MFT Server.
Minimum Requirements
- Java programming experience
- Java IDE
- JDK (Minimum requirements vary based on your version of JSCAPE MFT Server. Consult your documentation for details
- Experience creating Triggers in JSCAPE MFT Server
Introduction
The Triggers module in JSCAPE MFT Server comes with a comprehensive suite of 100+ built-in actions that can be used for executing everything from local operations (Copy File, Rename File, Zip Directory ...) to remote network operations (Trading Partner File Upload, Send Email, Twitter Update ...).
Utilizing these built-in actions one can automate most business processes, but in the rare event where an appropriate action does not exist there are two options available:
- Invoke an external command line process using the Run Process action
- Develop a custom action via the extensible action API provided by JSCAPE MFT Server.
While invoking an external command is often the quickest method to achieve your goal, assuming a command line utility exists, it depends on an external application and can lack the tight integration (i.e. visibility and error handling) realized when using an integrated custom action. The upfront development effort needed to create a custom action is minimal and once a custom action has been created it can be reused in any of your trigger definitions. Hurray for code re-use!
Getting Started
This tutorial will demonstrate how to create a custom trigger action in it's simplest form. Borrowing from the classic HelloWorld
examples of modern programming languages we will develop a HelloWorld
custom action. This action will have a single Greeting input property that will be printed to the Java System.out
output stream and log file upon execution. Before we fire up our IDE and start looking at source code it may be helpful to understand the basic components of a trigger action.
At it's core an action has one or more inputs, code execution and the execution result. The inputs to the action are populated at design-time when creating a trigger and may contain static or variable data that are executed upon at run-time.
Creating a custom action requires that we use a coding template that defines all of the input properties along with the code to be executed at run-time.
Coding Requirements
- In order to compile your custom action you must include all Java libraries found in the
libs
directory relative to your JSCAPE MFT Server installation in your IDE projectCLASSPATH
. - All custom actions must extend the abstract class
com.jscape.inet.mft.workflow.AbstractAction
and implement the abstract methods therein, namelygetDescription()
,getPropertyDescriptors()
andexecute()
.
Example Code
Code Analysis
- Lines 1 - 5: Necessary import statements.
- Line 6: Class definition. Must extend abstract class
com.jscape.inet.mft.workflow.AbstractAction
- Line 9: static variable
DESCRIPTION
used ingetDescription()
method and implemented in Lines 41 - 47. - Lines 10 - 12: Property descriptor used to define input properties used in executing action.
- Line 13: Variable for holding Greeting input property.
- Lines 16 - 21: Constructor for
HelloWorld
action invokes constructor of parent classAbstractAction
passing in location of inline help documentation properties. - Lines 25 - 37: Getter and setter methods for action properties.
- Lines 49 - 55: Implemented required
getPropertyDescriptors()
method. - Lines 61 - 64: Implemented required
execute()
method. - Line 63: Sets
resultMessage
variable of parent classAbstractAction
. The value of this variable will be printed in domain activity logs.
Defining Properties
JSCAPE MFT Server uses reflection on action classes to build the GUI screens used in collecting action inputs. This is convenient in that when creating actions you do not have to create separate GUI screens for collecting the data as these inputs are automatically generated. However, in order to generate these GUI panels there are some design rules that you must follow:
- Displayed action name is derived from it's class name. It should be mixed case with the first word of each internal word capitalized and be simple yet descriptive. For example, in the code example provided the
HelloWorld
class will be displayed as Hello World in the list of available actions in the Triggers module of JSCAPE MFT Server. - Exposed class variables must be primitives e.g.
String
,int
,long
,boolean
etc. - Exposed class variables must have corresponding getter and setter methods using Java naming conventions. For example, in the code example provided the
String
variablegreeting
has getter and setter methods ofgetGreeting
andsetGreeting
respectively. - You must implement the
getPropertyDescriptors()
method from the parentAbstractAction
class, returning acom.jscape.util.reflection.PropertyDescriptor[]
array that lists all the available inputs for your custom action.
When creating a PropertyDescriptor
instance the constructor used will define the input type, appearance and location of your custom action inputs in the GUI. See the JavaDoc API available in doc/api
directory relative to your JSCAPE MFT Server installation for a list of available constructors along with Lines 10 - 12 and Lines 49 - 55 in the supplied HelloWorld
example for details.
The HelloWorld
example has a single text field input parameter but there are a range of other input types that you can use when creating your own actions including MemoField
, BooleanField
, ChoiceField
and others. See the JavaDoc for the class com.jscape.util.reflection.FieldType
for a list of available input types.
Execution
This is where the magic happens. In the execute()
method of your AbstractAction
implementation add the code to execute when running this action. This is shown in Lines 61 - 64 of the supplied HelloWorld
source code example.
The signature of the execute()
method throws an Exception
. In the event that an exception is thrown the action will have a failed status, and conversely if no Exception
is thrown a successful status.
It is good coding practice to use a try/catch/finally
statement within your execute()
method to capture and re-throw any Exception
that may be thrown during execution and set the parent resultMessage
property to communicate any additional details back to Logging module in JSCAPE MFT Server and release any resources used during execution.
Documentation
Download HelloWorldHelp.properties
Like any good programmer you will want to document your custom action. Knowing how many dislike writing documentation we have made this process as painless as possible.
The first step is to document the purpose of this action. This is achieved by implementing the getDescription()
method and is shown in Lines 41 - 47 of the provided example. The value returned by getDescription()
will be shown in the inline help displayed in the Add Action dialog when creating/editing a trigger as well as in the Actions tab of the Triggers module.
The second and final step is to create a properties file that contains a list of all the available input parameters, their descriptions and and example inputs. To ensure this properties file is loaded when displaying your custom action override the base constructor for AbstractAction
passing in the location of the properties file. See Lines 16 - 21 of HelloWorld
class for an example.
See, I told you that wouldn't hurt.
Installation
For actions to be made available you must create a JAR archive that contains your actions and place it in the libs/actions
relative to your JSCAPE MFT Server installation. Any third party libraries that your action depends on should be placed in the libs
directory of your JSCAPE MFT Server installation. For your action to be recognized restart the JSCAPE MFT Server service.
To verify that your action has been successfully installed login to the administrative interface and navigate to the Triggers module for your domain. Click on the Actions tab to verify that your newly created action is visible.
Next Steps
Want to try this out?
Request a free, no-risk trial of JSCAPE and let our product experts support your next steps.