Sending Email with Java SMTP Library
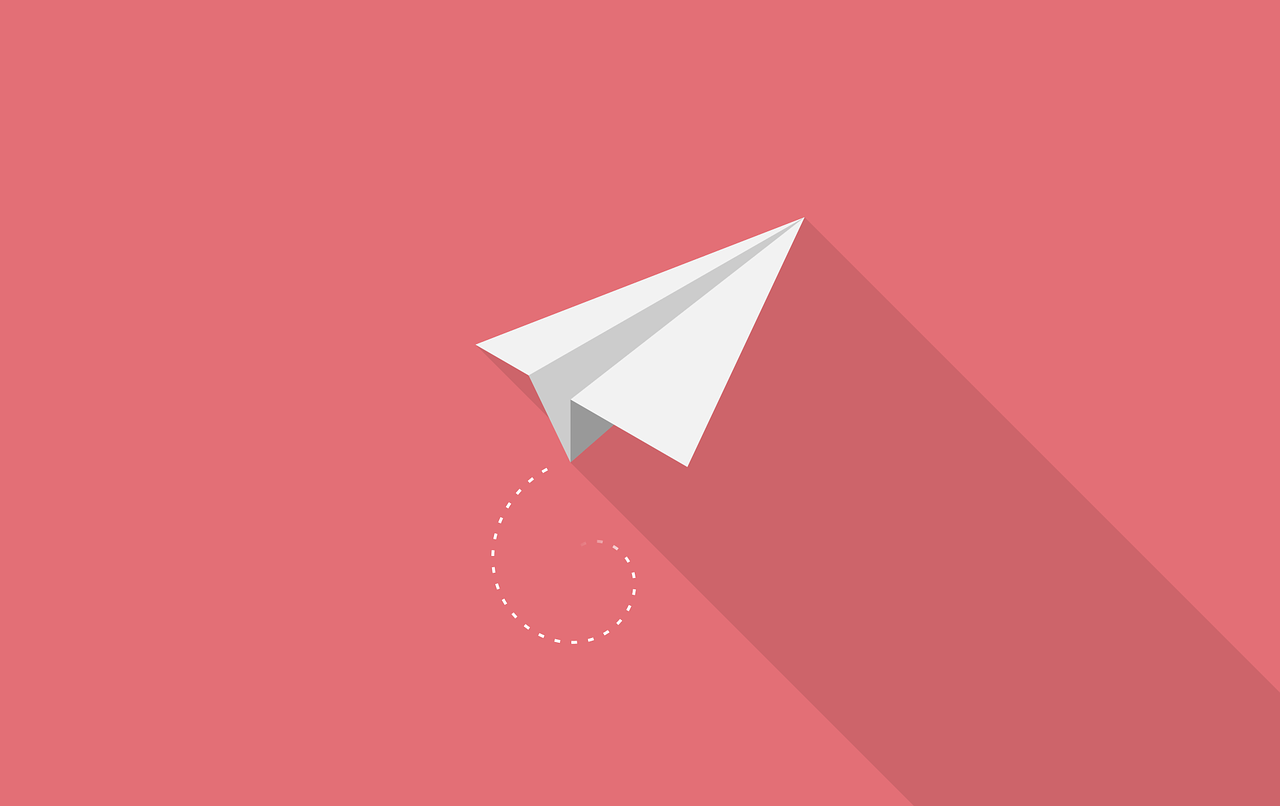
So let's say you have developed a marvelous herbal supplement that will help men grow larger ears, and you need to share this discovery with the world (or at least, that part of the world that is willing to send you $12.95 for your special mix of grass clippings from your backyard). You can use SMTP (Simple Mail Transfer Protocol) to route your message through the Internet, and the Secure iNet Factory library to help you write a Java program to do this.
This sample shows a simple mailer that will send a pre-defined message to an address you enter:
Sending Email with Java SMTP
import com.jscape.inet.smtp.*; import com.jscape.inet.email.*; import com.jscape.inet.mime.MimeException; import java.io.*; public class SMTPMailer extends SmtpAdapter { public void sendMessage(String toName) throws SmtpException, MimeException { // The SMTP host your email goes through. String SMTPHost = "smtp.somewebsite.com"; // The email address you want to use as "From". String fromName = "jdoakes@mailinator.com"; // The text of the email. String subject = "Women will love you for this!!!!"; String body = "Women love men who LISTEN. Make your "; body += "ears LARGER and MORE SENSITIVE with our "; body += "all-natural herbal supplement!"; // Create an Smtp object and add a Listener to catch status messages. Smtp smtp = new Smtp(SMTPHost); smtp.addSmtpListener(this); smtp.setDebug(true); // Connect to the server initialized above. smtp.connect(); // Create an Email with the properties set above. EmailMessage theEmail = new EmailMessage(); theEmail.setTo(toName); theEmail.setFrom(fromName); theEmail.setSubject(subject); theEmail.setBody(body); // Send the Email, then disconnect. smtp.send(theEmail); smtp.disconnect(); } // Capture and display connect events public void connected(SmtpConnectedEvent evt) { System.out.println("Connected to SMTP server: " + evt.getHostname()); } public void disconnected(SmtpDisconnectedEvent evt) { System.out.println("Disconnected from SMTP server: " + evt.getHostname()); } // For demo purposes, send this message to one address at a time. public static void main(String[] args) { String toName; try { BufferedReader reader = new BufferedReader(new InputStreamReader(System.in)); System.out.print("SMTP Mailer: Enter To address (e.g. recipient@domain.com): "); toName = reader.readLine(); SMTPMailer theMailer = new SMTPMailer(); theMailer.sendMessage(toName); } catch(Exception e) { e.printStackTrace(); } } }
Realistically, a class like this will only work if you have your own SMTP server on your network, which is configured to accept traffic only from your own computers. This server will often be called "smtp.yourwebsite.com" or "mail.yourwebsite.com" β ask your IT guy.
If your SMTP server is run by your ISP (Internet Service Provider) or anyone external, it will probably require authentication before you can send email out. Any SMTP server that does not do this is just asking to be abused by spammers. (That's not you, of course.) You may have to chase around for a while to find the name and password for your SMTP server, which is often obscure.
For example, Google allows you to send email from your GMail account, provided that you give it the right authentication, which is based on your Google login. See Sending Email using Java GMail and SMTP for a discussion.
By the way, if you're not familiar with it, you will find Mailinator.com a very handy free tool for testing email applications.